In this article, we are going to see basic knowledge of OOP Concepts.
Understanding OOP concepts help us to make decisions about how you should design an application and what language to be used.
key concepts of OOPs are inheritance, abstraction, encapsulation, and polymorphism.
What is OOP?
OOPS stand for Object-Oriented Programming.
- It is faster and easier to execute.
- It provides a proper structure for the programs.
- It helps to keep the code DRY Don’t Repeat Yourself, and makes the code easier to maintain, modify and debug.
- With the help of OOPs, it is possible to create full reusable applications with less code and shorter development time.
What are Classes and Objects?
An object is an instance of the class.
An object is a bunch of relative variables and methods.
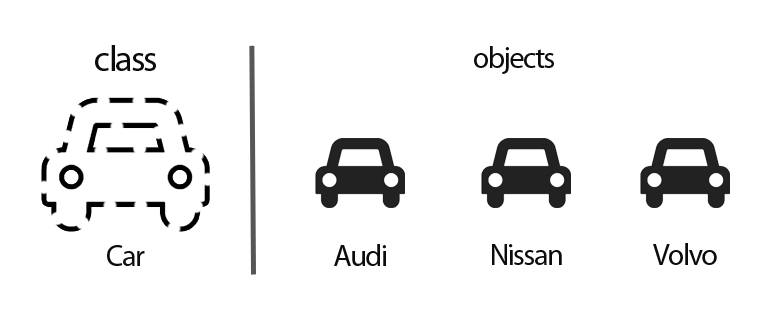
Objects are created from a class blueprint, which defines the data and behavior of all instances of that type.
Class Student { //fields //variables, //Methods, //properties, }
Here is an example of an object.
Student studentObject = new Student();
If An object is created with help of a new operator as you can see above, memory is allocated for the class in the heap, the object is called an instance.
An object is created without the new operator, memory will not be allocated in the heap, It means an instance will not be created and the object in the stack contains the null value.
Abstraction
Abstraction is a process to hide essential features without describing any background details.
Abstraction is important because it can hide unnecessary details from reference objects.
Abstraction lets you focus on what an object does Instead of showing how an object is represented or how it works. Therefore, data abstraction is often used for managing large and complex programs.
In C# abstraction is achieved with the help of Abstract classes.
Abstract Classes
- An abstract class is declared with the help of an abstract keyword.
- In C#, you cannot use the abstract class directly with the new operator.
- The class that contains the abstract keyword with some of its methods is known as an Abstract Base Class.
- The class that contains the abstract keyword with all of its methods is known as pure Abstract Base Class.
- We can not declare abstract methods outside the abstract class.
- We can not declare an abstract class as Sealed Class.
namespace TheCodeHubsExample { abstract class Pet { public abstract void petVoice(); } class Cat : Pet { public override void petVoice() { Console.WriteLine("The cat says: meow meow"); } } class Program { static void Main(string[] args) { Cat catObj = new Cat(); catObj.petVoice(); } } }
Encapsulation
Encapsulation means wrapping up data in a single unit. It is the mechanism that binds member function and data member into a single class.
In other words, encapsulation is a protective shield that prevents the data from being accessed outside of this shield.
The names encapsulation comes from the fact that this class encapsulates the set of methods, properties, and attributes.
using System; using System.Text; namespace EncapsulationExample { class User { public string Name; public string Email; } class Program { static void Main(string[] args) { User u = new User(); // set accessor will invoke u.Name = "TheCodeHubs"; // set accessor will invoke u.Email = "thecodehubs@gmail.com"; // get accessor will invoke Console.WriteLine("Name: " + u.Name); // get accessor will invoke Console.WriteLine("Email: " + u.Email); } } }
- Data Hiding: The user has no idea about the inner implementation of the class. They only know that we are passing the values to accessors and variables are getting initialized to that value.
- Flexibility Increases: We can make the variables of the class read-only or write-only depending on our requirement. If we wish to make the variables read-only then we have to only use Get Accessor in the code. If we wish to make the variables write-only then we have to only use Set Accessor.
- Reusability: Encapsulation also improves the reusability of code and easy to do changes with new requirements.
- Testing code is easy: Encapsulated code is easy to test for unit testing.
Polymorphism
Polymorphism means one name, many forms.
In other words, we can say one function can behave in different ways.
There are two types of polymorphism in C#.
- Static polymorphism.
- Dynamic polymorphism.
Static polymorphism.
static polymorphism is achieved with the help of function overloading and operator overloading in C#. It is also known as early binding and compile-time polymorphism.
Dynamic polymorphism.
Dynamic polymorphism is achieved with the help of Method overriding which is also known as late binding and runtime polymorphism.
Inheritance
Inheritance is a process in which one object acquires all the properties and behaviors of its parent object automatically. In such a way, you can reuse, or modify the attributes and behaviors which is defined in other class.
The class which inherits the members of another class is called the derived class and the class whose members are inherited is called the base class. The derived class is the child class for the base class.
class BaseClass { } class DerivedClass : BaseClass { }
That’s it.
Also, Check File Upload Using FTP In .Net Core 6.0